- Blog Categories
- Software Development
- Data Science
- AI/ML
- Marketing
- General
- MBA
- Management
- Legal
- Software Development Projects and Ideas
- 12 Computer Science Project Ideas
- 28 Beginner Software Projects
- Top 10 Engineering Project Ideas
- Top 10 Easy Final Year Projects
- Top 10 Mini Projects for Engineers
- 25 Best Django Project Ideas
- Top 20 MERN Stack Project Ideas
- Top 12 Real Time Projects
- Top 6 Major CSE Projects
- 12 Robotics Projects for All Levels
- Java Programming Concepts
- Abstract Class in Java and Methods
- Constructor Overloading in Java
- StringBuffer vs StringBuilder
- Java Identifiers: Syntax & Examples
- Types of Variables in Java Explained
- Composition in Java: Examples
- Append in Java: Implementation
- Loose Coupling vs Tight Coupling
- Integrity Constraints in DBMS
- Different Types of Operators Explained
- Career and Interview Preparation in IT
- Top 14 IT Courses for Jobs
- Top 20 Highest Paying Languages
- 23 Top CS Interview Q&A
- Best IT Jobs without Coding
- Software Engineer Salary in India
- 44 Agile Methodology Interview Q&A
- 10 Software Engineering Challenges
- Top 15 Tech's Daily Life Impact
- 10 Best Backends for React
- Cloud Computing Reference Models
- Web Development and Security
- Find Installed NPM Version
- Install Specific NPM Package Version
- Make API Calls in Angular
- Install Bootstrap in Angular
- Use Axios in React: Guide
- StrictMode in React: Usage
- 75 Cyber Security Research Topics
- Top 7 Languages for Ethical Hacking
- Top 20 Docker Commands
- Advantages of OOP
- Data Science Projects and Applications
- 42 Python Project Ideas for Beginners
- 13 Data Science Project Ideas
- 13 Data Structure Project Ideas
- 12 Real-World Python Applications
- Python Banking Project
- Data Science Course Eligibility
- Association Rule Mining Overview
- Cluster Analysis in Data Mining
- Classification in Data Mining
- KDD Process in Data Mining
- Data Structures and Algorithms
- Binary Tree Types Explained
- Binary Search Algorithm
- Sorting in Data Structure
- Binary Tree in Data Structure
- Binary Tree vs Binary Search Tree
- Recursion in Data Structure
- Data Structure Search Methods: Explained
- Binary Tree Interview Q&A
- Linear vs Binary Search
- Priority Queue Overview
- Python Programming and Tools
- Top 30 Python Pattern Programs
- List vs Tuple
- Python Free Online Course
- Method Overriding in Python
- Top 21 Python Developer Skills
- Reverse a Number in Python
- Switch Case Functions in Python
- Info Retrieval System Overview
- Reverse a Number in Python
- Real-World Python Applications
- Data Science Careers and Comparisons
- Data Analyst Salary in India
- Data Scientist Salary in India
- Free Excel Certification Course
- Actuary Salary in India
- Data Analyst Interview Guide
- Pandas Interview Guide
- Tableau Filters Explained
- Data Mining Techniques Overview
- Data Analytics Lifecycle Phases
- Data Science Vs Analytics Comparison
- Artificial Intelligence and Machine Learning Projects
- Exciting IoT Project Ideas
- 16 Exciting AI Project Ideas
- 45+ Interesting ML Project Ideas
- Exciting Deep Learning Projects
- 12 Intriguing Linear Regression Projects
- 13 Neural Network Projects
- 5 Exciting Image Processing Projects
- Top 8 Thrilling AWS Projects
- 12 Engaging AI Projects in Python
- NLP Projects for Beginners
- Concepts and Algorithms in AIML
- Basic CNN Architecture Explained
- 6 Types of Regression Models
- Data Preprocessing Steps
- Bagging vs Boosting in ML
- Multinomial Naive Bayes Overview
- Bayesian Network Example
- Bayes Theorem Guide
- Top 10 Dimensionality Reduction Techniques
- Neural Network Step-by-Step Guide
- Technical Guides and Comparisons
- Make a Chatbot in Python
- Compute Square Roots in Python
- Permutation vs Combination
- Image Segmentation Techniques
- Generative AI vs Traditional AI
- AI vs Human Intelligence
- Random Forest vs Decision Tree
- Neural Network Overview
- Perceptron Learning Algorithm
- Selection Sort Algorithm
- Career and Practical Applications in AIML
- AI Salary in India Overview
- Biological Neural Network Basics
- Top 10 AI Challenges
- Production System in AI
- Top 8 Raspberry Pi Alternatives
- Top 8 Open Source Projects
- 14 Raspberry Pi Project Ideas
- 15 MATLAB Project Ideas
- Top 10 Python NLP Libraries
- Naive Bayes Explained
- Digital Marketing Projects and Strategies
- 10 Best Digital Marketing Projects
- 17 Fun Social Media Projects
- Top 6 SEO Project Ideas
- Digital Marketing Case Studies
- Coca-Cola Marketing Strategy
- Nestle Marketing Strategy Analysis
- Zomato Marketing Strategy
- Monetize Instagram Guide
- Become a Successful Instagram Influencer
- 8 Best Lead Generation Techniques
- Digital Marketing Careers and Salaries
- Digital Marketing Salary in India
- Top 10 Highest Paying Marketing Jobs
- Highest Paying Digital Marketing Jobs
- SEO Salary in India
- Content Writer Salary Guide
- Digital Marketing Executive Roles
- Career in Digital Marketing Guide
- Future of Digital Marketing
- MBA in Digital Marketing Overview
- Digital Marketing Techniques and Channels
- 9 Types of Digital Marketing Channels
- Top 10 Benefits of Marketing Branding
- 100 Best YouTube Channel Ideas
- YouTube Earnings in India
- 7 Reasons to Study Digital Marketing
- Top 10 Digital Marketing Objectives
- 10 Best Digital Marketing Blogs
- Top 5 Industries Using Digital Marketing
- Growth of Digital Marketing in India
- Top Career Options in Marketing
- Interview Preparation and Skills
- 73 Google Analytics Interview Q&A
- 56 Social Media Marketing Q&A
- 78 Google AdWords Interview Q&A
- Top 133 SEO Interview Q&A
- 27+ Digital Marketing Q&A
- Digital Marketing Free Course
- Top 9 Skills for PPC Analysts
- Movies with Successful Social Media Campaigns
- Marketing Communication Steps
- Top 10 Reasons to Be an Affiliate Marketer
- Career Options and Paths
- Top 25 Highest Paying Jobs India
- Top 25 Highest Paying Jobs World
- Top 10 Highest Paid Commerce Job
- Career Options After 12th Arts
- Top 7 Commerce Courses Without Maths
- Top 7 Career Options After PCB
- Best Career Options for Commerce
- Career Options After 12th CS
- Top 10 Career Options After 10th
- 8 Best Career Options After BA
- Projects and Academic Pursuits
- 17 Exciting Final Year Projects
- Top 12 Commerce Project Topics
- Top 13 BCA Project Ideas
- Career Options After 12th Science
- Top 15 CS Jobs in India
- 12 Best Career Options After M.Com
- 9 Best Career Options After B.Sc
- 7 Best Career Options After BCA
- 22 Best Career Options After MCA
- 16 Top Career Options After CE
- Courses and Certifications
- 10 Best Job-Oriented Courses
- Best Online Computer Courses
- Top 15 Trending Online Courses
- Top 19 High Salary Certificate Courses
- 21 Best Programming Courses for Jobs
- What is SGPA? Convert to CGPA
- GPA to Percentage Calculator
- Highest Salary Engineering Stream
- 15 Top Career Options After Engineering
- 6 Top Career Options After BBA
- Job Market and Interview Preparation
- Why Should You Be Hired: 5 Answers
- Top 10 Future Career Options
- Top 15 Highest Paid IT Jobs India
- 5 Common Guesstimate Interview Q&A
- Average CEO Salary: Top Paid CEOs
- Career Options in Political Science
- Top 15 Highest Paying Non-IT Jobs
- Cover Letter Examples for Jobs
- Top 5 Highest Paying Freelance Jobs
- Top 10 Highest Paying Companies India
- Career Options and Paths After MBA
- 20 Best Careers After B.Com
- Career Options After MBA Marketing
- Top 14 Careers After MBA In HR
- Top 10 Highest Paying HR Jobs India
- How to Become an Investment Banker
- Career Options After MBA - High Paying
- Scope of MBA in Operations Management
- Best MBA for Working Professionals India
- MBA After BA - Is It Right For You?
- Best Online MBA Courses India
- MBA Project Ideas and Topics
- 11 Exciting MBA HR Project Ideas
- Top 15 MBA Project Ideas
- 18 Exciting MBA Marketing Projects
- MBA Project Ideas: Consumer Behavior
- What is Brand Management?
- What is Holistic Marketing?
- What is Green Marketing?
- Intro to Organizational Behavior Model
- Tech Skills Every MBA Should Learn
- Most Demanding Short Term Courses MBA
- MBA Salary, Resume, and Skills
- MBA Salary in India
- HR Salary in India
- Investment Banker Salary India
- MBA Resume Samples
- Sample SOP for MBA
- Sample SOP for Internship
- 7 Ways MBA Helps Your Career
- Must-have Skills in Sales Career
- 8 Skills MBA Helps You Improve
- Top 20+ SAP FICO Interview Q&A
- MBA Specializations and Comparative Guides
- Why MBA After B.Tech? 5 Reasons
- How to Answer 'Why MBA After Engineering?'
- Why MBA in Finance
- MBA After BSc: 10 Reasons
- Which MBA Specialization to choose?
- Top 10 MBA Specializations
- MBA vs Masters: Which to Choose?
- Benefits of MBA After CA
- 5 Steps to Management Consultant
- 37 Must-Read HR Interview Q&A
- Fundamentals and Theories of Management
- What is Management? Objectives & Functions
- Nature and Scope of Management
- Decision Making in Management
- Management Process: Definition & Functions
- Importance of Management
- What are Motivation Theories?
- Tools of Financial Statement Analysis
- Negotiation Skills: Definition & Benefits
- Career Development in HRM
- Top 20 Must-Have HRM Policies
- Project and Supply Chain Management
- Top 20 Project Management Case Studies
- 10 Innovative Supply Chain Projects
- Latest Management Project Topics
- 10 Project Management Project Ideas
- 6 Types of Supply Chain Models
- Top 10 Advantages of SCM
- Top 10 Supply Chain Books
- What is Project Description?
- Top 10 Project Management Companies
- Best Project Management Courses Online
- Salaries and Career Paths in Management
- Project Manager Salary in India
- Average Product Manager Salary India
- Supply Chain Management Salary India
- Salary After BBA in India
- PGDM Salary in India
- Top 7 Career Options in Management
- CSPO Certification Cost
- Why Choose Product Management?
- Product Management in Pharma
- Product Design in Operations Management
- Industry-Specific Management and Case Studies
- Amazon Business Case Study
- Service Delivery Manager Job
- Product Management Examples
- Product Management in Automobiles
- Product Management in Banking
- Sample SOP for Business Management
- Video Game Design Components
- Top 5 Business Courses India
- Free Management Online Course
- SCM Interview Q&A
- Fundamentals and Types of Law
- Acceptance in Contract Law
- Offer in Contract Law
- 9 Types of Evidence
- Types of Law in India
- Introduction to Contract Law
- Negotiable Instrument Act
- Corporate Tax Basics
- Intellectual Property Law
- Workmen Compensation Explained
- Lawyer vs Advocate Difference
- Law Education and Courses
- LLM Subjects & Syllabus
- Corporate Law Subjects
- LLM Course Duration
- Top 10 Online LLM Courses
- Online LLM Degree
- Step-by-Step Guide to Studying Law
- Top 5 Law Books to Read
- Why Legal Studies?
- Pursuing a Career in Law
- How to Become Lawyer in India
- Career Options and Salaries in Law
- Career Options in Law India
- Corporate Lawyer Salary India
- How To Become a Corporate Lawyer
- Career in Law: Starting, Salary
- Career Opportunities: Corporate Law
- Business Lawyer: Role & Salary Info
- Average Lawyer Salary India
- Top Career Options for Lawyers
- Types of Lawyers in India
- Steps to Become SC Lawyer in India
- Tutorials
- Software Tutorials
- C Tutorials
- Recursion in C: Fibonacci Series
- Checking String Palindromes in C
- Prime Number Program in C
- Implementing Square Root in C
- Matrix Multiplication in C
- Understanding Double Data Type
- Factorial of a Number in C
- Structure of a C Program
- Building a Calculator Program in C
- Compiling C Programs on Linux
- Java Tutorials
- Handling String Input in Java
- Determining Even and Odd Numbers
- Prime Number Checker
- Sorting a String
- User-Defined Exceptions
- Understanding the Thread Life Cycle
- Swapping Two Numbers
- Using Final Classes
- Area of a Triangle
- Skills
- Explore Skills
- Management Skills
- Software Engineering
- JavaScript
- Data Structure
- React.js
- Core Java
- Node.js
- Blockchain
- SQL
- Full stack development
- Devops
- NFT
- BigData
- Cyber Security
- Cloud Computing
- Database Design with MySQL
- Cryptocurrency
- Python
- Digital Marketings
- Advertising
- Influencer Marketing
- Performance Marketing
- Search Engine Marketing
- Email Marketing
- Content Marketing
- Social Media Marketing
- Display Advertising
- Marketing Analytics
- Web Analytics
- Affiliate Marketing
- MBA
- MBA in Finance
- MBA in HR
- MBA in Marketing
- MBA in Business Analytics
- MBA in Operations Management
- MBA in International Business
- MBA in Information Technology
- MBA in Healthcare Management
- MBA In General Management
- MBA in Agriculture
- MBA in Supply Chain Management
- MBA in Entrepreneurship
- MBA in Project Management
- Management Program
- Consumer Behaviour
- Supply Chain Management
- Financial Analytics
- Introduction to Fintech
- Introduction to HR Analytics
- Fundamentals of Communication
- Art of Effective Communication
- Introduction to Research Methodology
- Mastering Sales Technique
- Business Communication
- Fundamentals of Journalism
- Economics Masterclass
- Free Courses
- Home
- Blog
- Data Science
- LOC vs ILOC Pandas: Understanding the Key Differences, Similarities, and More
LOC vs ILOC Pandas: Understanding the Key Differences, Similarities, and More
Updated on Jan 20, 2025 | 11 min read
Share:
Table of Contents
In Pandas, loc allows you to access data using row and column labels (names), while iloc selects data based on the integer positions of rows and columns.
Understanding the difference between loc vs iloc Pandas is particularly important in data science tasks like feature engineering, filtering data, or performing operations on large datasets.
What is loc in Pandas? Examples and Use Cases
In Pandas, loc is a label-based data selection method that allows you to access rows and columns using their labels or index names.
loc is used when you want to retrieve specific data from a DataFrame based on the index labels and column names. loc ensures accurate selection based on labels.
Here’s the syntax of .loc in Python.
df.loc[rows, columns]
Where,
rows specifies the label(s) of the rows to select.
columns specifies the label(s) of the columns to select.
To understand the implementation of loc using the above syntax, check out the following example, including its different use cases.
loc in Pandas Using Example
The use of loc in Pandas can be better understood through an example, where you can perform various operations using its syntax.
Let's begin by creating a DataFrame and then use loc to access specific data.
import pandas as pd
# Sample DataFrame with names
data = {
'Name': ['Arjun', 'Priya', 'Rajesh', 'Sita'],
'Age': [25, 28, 22, 30],
'City': ['Mumbai', 'Delhi', 'Bengaluru', 'Chennai']
}
df = pd.DataFrame(data)
df.set_index('Name', inplace=True)
print(df)
Output:
Age City
Name
Arjun 25 Mumbai
Priya 28 Delhi
Rajesh 22 Bengaluru
Sita 30 Chennai
Now that you’ve created a sample DataFrame, let’s use loc to perform various operations such as selecting a particular row or modifying data.
1. Selecting a row by label
You can access specific rows by name (like 'Arjun', 'Priya') rather than by numeric index positions. In case labels are missing, loc raises a KeyError because it requires explicit row and column labels to access data.
# Select row for 'Priya'
print(df.loc['Priya'])
Output:
Age 28
City Delhi
Name: Priya, dtype: object
2. Selecting specific rows and columns
Use loc to select both rows and columns at the same time, like getting the 'Age' and 'City' for specific names.
# Select Age and City for 'Arjun' and 'Sita'
print(df.loc[['Arjun', 'Sita'], ['Age', 'City']])
Output:
Age City
Name
Arjun 25 Mumbai
Sita 30 Chennai
3. Conditional selection
You can filter data using conditions like df['Age'] > 25 to get only those people whose age is greater than 25.
# Select rows where Age is greater than 25
print(df.loc[df['Age'] > 25])
Output:
Age City
Name
Priya 28 Delhi
Sita 30 Chennai
4. Setting values using loc
Using loc, you can update values for specific rows and columns, which is useful for modifying individual entries in the DataFrame. The use of loc for this task ensures readability and accuracy when working with labeled indices.
# Modify the age for Rajesh to 26
df.loc['Rajesh', 'Age'] = 26
print(df.loc['Rajesh'])
Output:
Age 26
City Bengaluru
Name: Rajesh, dtype: object
Updated DataFrame:
Age City
Name
Arjun 25 Mumbai
Priya 28 Delhi
Rajesh 26 Bengaluru
Sita 30 Chennai
5. Selecting a range of rows by label
Using loc, you can easily select a range of rows using the labels, such as from 'Rajesh' to 'Sita'.
# Select rows from 'Rajesh' to 'Sita' inclusively
print(df.loc['Rajesh':'Sita'])
Output:
Age City
Name
Rajesh 22 Bengaluru
Sita 30 Chennai
Now that you have discovered how loc allows you to access rows and columns using their labels, let’s check out how you can use positional-based indexing to access elements.
What is iloc in Python? Examples and Applications
In Pandas, iloc is an integer-location-based indexing method that selects rows and columns from a DataFrame using their integer positions, not by their labels or names.
Here’s the syntax for using iloc in Pandas.
df.iloc[rows, columns]
Where,
rows specifies the integer position(s) of the rows to select.
columns specifies the integer position(s) of the columns to select.
Learn the basics of Python programming and explore its applications for tasks such as data analysis. Join the Learn Basic Python Programming course.
To understand how iloc is used to access elements in a particular row or column, let’s explore a particular example and then consider different use cases.
iloc in Pandas Using Example
The first step is to create a sample DataFrame, which contains the names, ages and cities as data.
Here’s a code snippet for creating the DataFrame.
import pandas as pd
# Sample DataFrame with Indian names
data = {
'Name': ['Arjun', 'Priya', 'Rajesh', 'Sita'],
'Age': [25, 28, 22, 30],
'City': ['Mumbai', 'Delhi', 'Bengaluru', 'Chennai']
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age City
0 Arjun 25 Mumbai
1 Priya 28 Delhi
2 Rajesh 22 Bengaluru
3 Sita 30 Chennai
Now that you’ve created a sample dataset, let’s understand how to use iloc for specific use cases, such as selecting rows, modifying data, or slicing the dataset.
1. Selecting a row by integer position
iloc is particularly useful when you need to access rows by their position in the DataFrame.
# Select the second row using the indexing method (index 1)
print(df.iloc[1])
Output:
Name Priya
Age 28
City Delhi
Name: 1, dtype: object
2. Selecting specific rows and columns
Just like rows, columns can also be accessed using their integer positions.
# Select the Age and City columns for the first two rows (index 0 and 1)
print(df.iloc[0:2, [1, 2]])
Output:
Age City
0 25 Mumbai
1 28 Delhi
3. Using negative indices
Similar to Python lists, you can use negative indices with iloc.
# Select the last row using position-based negative indexing(using -1 to get the last position)
print(df.iloc[-1])
Output:
Name Sita
Age 30
City Chennai
Name: 3, dtype: object
4. Selecting a single element
You can use iloc to access a specific cell by passing the row index and column index.
# Select the element from the second row and the third column (Age for 'Priya')
print(df.iloc[1, 1])
Output:
28
5. Slicing rows
You can slice rows using iloc, just like list slicing in Python. For example, df.iloc[0:3] will return the first three rows.
# Implement slicing by selecting the first three rows
print(df.iloc[:3])
Output:
Name Age City
0 Arjun 25 Mumbai
1 Priya 28 Delhi
2 Rajesh 22 Bengaluru
6. Modify Data
You can also modify specific rows and columns in the DataFrame using integer positions with iloc.
# Modify data of Arjun's age and Rajesh's city using .iloc
df.iloc[0, 1] = 26 # Changing 'Age' of Arjun (first row) to 26
df.iloc[2, 2] = 'Hyderabad' # Changing 'City' of Rajesh (third row) to 'Hyderabad'
print(df)
Output:
Name Age City
CustomerID
C001 Arjun 26 Mumbai
C002 Priya 28 Delhi
C003 Rajesh 22 Hyderabad
C004 Sita 30 Chennai
Also Read: Pandas Cheat Sheet in Python for Data Science: Complete List for 2025
Now that you’ve seen how to use loc and iloc to access elements in a particular row or column, let’s understand the difference between loc and iloc in Pandas.
upGrad’s Exclusive Data Science Webinar for you –
Watch our Webinar on How to Build Digital & Data Mindset?
Difference Between loc and iloc in Pandas: Key Differences Explained
The difference between loc and iloc is mainly based on the way elements are selected. While loc supports selection based on labels, iloc uses indices for selection.
Here are the loc vs iloc differences.
Parameter | loc | iloc |
Selection Type | Label-based selection | Integer-based selection |
Indexing Type | Works with row/column labels or index names | Works with integer indices (zero-based) |
Inclusion of End in Slicing | The end index is included in slicing. | The end index is excluded in slicing. |
Error Handling Error Type | Raises KeyError when an invalid label is used. | Raises IndexError when an invalid position is used. |
Use of Labels | Uses explicit labels (e.g., 'Rajesh', 'Age') | Uses integer positions (e.g., 0, 1, 2) |
Slicing | Can slice by label (e.g., df.loc['a':'c']) | Can slice by position (e.g., df.iloc[0:3]) |
Modifying Data | Data can be modified by label | Data can be modified by position |
Support for Boolean Indexing | Supports Boolean indexing directly. (e.g., df.loc[df['Age'] > 25]) | Does not support Boolean indexing. |
Also Read: Mastering Pandas: Important Pandas Functions For Your Next Project
While LOC and ILOC differ in their methods for selecting elements, they share similarities in selecting non-contiguous rows and columns. Let’s explore these similarities in more detail.
loc vs iloc Pandas: Similarities
The loc and iloc methods show similarities when it comes to selecting non-contiguous rows and columns or handling out-of-bound errors.
Here are the similarities between loc vs iloc.
- Simultaneous Selection of Row and Column
Both loc and iloc allow you to select both rows and columns at the same time, providing flexibility in data retrieval. This is especially useful in scenarios where you need to access a specific data point from a large dataset.
Example: For loc, you can use df.loc['row1', 'col1'] to select a specific value using label-based indexing. iloc uses df.iloc[0, 0] to select a specific value using position-based indexing.
- Handling of Out-of-Bounds Errors
Both loc and iloc throw similar error types when invalid rows or columns are selected. This is useful during debugging when you mistakenly reference non-existent rows/columns.
Example: The loc raises KeyError when an invalid label is provided, while iloc raises IndexError when the out-of-bound index is accessed.
- Accessing Single and Multiple Cells
Both loc and iloc enable the selection of both single values and multiple values from DataFrames. This will help streamline data extraction when working with subsets of a dataset.
Example: loc uses df.loc['row1', ['col1', 'col2']] to select multiple columns for a specific row. The iloc uses df.iloc[0, [0, 1]] to select multiple columns for the first row.
- Selecting Multiple Rows and Columns
Both loc and iloc support selecting multiple rows and columns by providing a list of label positions. This is beneficial if you need to extract or analyze subsets of the data for modeling or visualization.
Example: loc uses df.loc[['row1', 'row2'], ['col1', 'col2']] to select multiple rows and columns, whereas iloc uses df.iloc[[0, 1], [0, 2]] to perform the same.
- Supports Slicing
Both loc and iloc allow slicing to be performed, allowing you to select a range of rows and columns. It is useful in cases like analyzing data within a specific range or time period.
Example: loc uses df.loc['row1':'row3'] to select all rows from 'row1' to 'row3', including both endpoints. iloc performs slicing with integer positions, where df.iloc[0:3] selects the first three rows but excludes the endpoints.
While there are differences, loc and iloc provide similar functionality for accessing, modifying, and slicing data in Pandas DataFrames. To understand the behavior of these methods, let’s explore a real-world example.
In-Depth Example: loc and iloc in Pandas
For an in-depth understanding of loc and iloc in Pandas, let’s perform operations on a Telco Customer dataset, which can be sourced from platforms like Kaggle.
Here’s a step-by-step process to understand the working of loc and iloc for the telecom customer dataset.
1. Adding the Dataset to the DataFrame
The first step is to load the Telco customer dataset into a Pandas DataFrame. You can either create a simple DataFrame from a dictionary or load it from an external CSV or Excel file. In this case, you will create the dataset manually.
import pandas as pd
# Sample Telco Customer DataFrame
data = {
'CustomerID': ['C001', 'C002', 'C003', 'C004'],
'Name': ['Rahul', 'Priya', 'Amit', 'Anjali'],
'Age': [28, 34, 22, 45],
'City': ['Delhi', 'Mumbai', 'Bengaluru', 'Chennai'],
'Subscription': ['Basic', 'Premium', 'Basic', 'Premium']
}
# Create a DataFrame
df = pd.DataFrame(data)
# Set 'CustomerID' as the index for better readability
df.set_index('CustomerID', inplace=True)
# Display the DataFrame
print(df)
Output:
Name Age City Subscription
CustomerID
C001 Rahul 28 Delhi Basic
C002 Priya 34 Mumbai Premium
C003 Amit 22 Bengaluru Basic
C004 Anjali 45 Chennai Premium
Also Read: Pandas Dataframe Astype: Syntax, Data Types, Creating Dataframe
2. Selecting a specific row
The loc selects rows based on their labels (names), while the iloc uses the index to select. Let’s see how loc and iloc is used to select data for different cases.
- Select a specific row using loc
# Select a specific row using loc (based on CustomerID)
loc_result = df.loc['C003']
print("\nSelected Row using loc (CustomerID = C003):")
print(loc_result)
Output:
Selected Row using loc (CustomerID = C003):
Name Amit
Age 22
City Bengaluru
Subscription Basic
Name: C003, dtype: object
Here, loc selects the row corresponding to customer 'C003' using the label.
- Select specific rows using iloc
# Select a specific row using iloc (based on index position, 2nd index)
iloc_result = df.iloc[2]
print("\nSelected Row using iloc (Index position = 2):")
print(iloc_result)
Output:
Selected Row using iloc (Index position = 2):
Name Amit
Age 22
City Bengaluru
Subscription Basic
Name: C003, dtype: object
Here, iloc selects the rows for 'C003' based on index position 2.
3. Difference between loc and iloc for this example
To understand how loc and iloc work, you’ll perform the same operation using both these methods.
- Using loc to select 'Name' and 'City' for 'C003':
loc_result = df.loc['C003', ['Name', 'City']]
print(loc_result)
Output:
Name Amit
City Bengaluru
Name: C003, dtype: object
- Using iloc to select 'Name' and 'City' for the third row
iloc_result = df.iloc[2, [0, 3]]
print(iloc_result)
Output:
Name Amit
City Bengaluru
Name: C003, dtype: object
You can observe that loc uses the label 'C003' to select data, while iloc uses the integer position (2) to select the third row. To access columns, loc uses their labels ('Name' and 'City'), while iloc uses the column positions (0 for 'Name' and 3 for 'City').
Now that you’ve understood the workings of loc and iloc through a practical example, let’s now explore ways to boost your knowledge in this field.
Transform Your Career with upGrad’s Expert-Led Programs
In data analytics and machine learning, loc and iloc are essential tools for selecting and manipulating data within Pandas DataFrames. upGrad’s specialized courses will provide you with hands-on learning opportunities to learn tools like Python, Pandas, loc, iloc, and more to solve real-world challenges.
Here are some courses that can help you learn data handling:
Unlock the power of data with our popular Data Science courses, designed to make you proficient in analytics, machine learning, and big data!
Explore our Popular Data Science Courses
Elevate your career by learning essential Data Science skills such as statistical modeling, big data processing, predictive analytics, and SQL!
Top Data Science Skills to Learn
Stay informed and inspired with our popular Data Science articles, offering expert insights, trends, and practical tips for aspiring data professionals
Read our popular Data Science Articles
Frequently Asked Questions (FAQs)
1. What is iloc in Python?
2. Is loc inclusive?
3. What is df in Python?
4. How to remove duplicates in Pandas?
5. What is the difference between loc vs iloc?
6. What is the difference between a dataframe and a series?
7. What happens if labels are missing when using loc?
8. Can iloc handle mixed indices?
9. What is slicing in Python?
10. What is indexing in Python?
Get Free Consultation
By submitting, I accept the T&C and
Privacy Policy
Start Your Career in Data Science Today
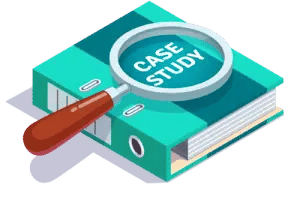
Top Resources