- Blog Categories
- Software Development
- Data Science
- AI/ML
- Marketing
- General
- MBA
- Management
- Legal
- Software Development Projects and Ideas
- 12 Computer Science Project Ideas
- 28 Beginner Software Projects
- Top 10 Engineering Project Ideas
- Top 10 Easy Final Year Projects
- Top 10 Mini Projects for Engineers
- 25 Best Django Project Ideas
- Top 20 MERN Stack Project Ideas
- Top 12 Real Time Projects
- Top 6 Major CSE Projects
- 12 Robotics Projects for All Levels
- Java Programming Concepts
- Abstract Class in Java and Methods
- Constructor Overloading in Java
- StringBuffer vs StringBuilder
- Java Identifiers: Syntax & Examples
- Types of Variables in Java Explained
- Composition in Java: Examples
- Append in Java: Implementation
- Loose Coupling vs Tight Coupling
- Integrity Constraints in DBMS
- Different Types of Operators Explained
- Career and Interview Preparation in IT
- Top 14 IT Courses for Jobs
- Top 20 Highest Paying Languages
- 23 Top CS Interview Q&A
- Best IT Jobs without Coding
- Software Engineer Salary in India
- 44 Agile Methodology Interview Q&A
- 10 Software Engineering Challenges
- Top 15 Tech's Daily Life Impact
- 10 Best Backends for React
- Cloud Computing Reference Models
- Web Development and Security
- Find Installed NPM Version
- Install Specific NPM Package Version
- Make API Calls in Angular
- Install Bootstrap in Angular
- Use Axios in React: Guide
- StrictMode in React: Usage
- 75 Cyber Security Research Topics
- Top 7 Languages for Ethical Hacking
- Top 20 Docker Commands
- Advantages of OOP
- Data Science Projects and Applications
- 42 Python Project Ideas for Beginners
- 13 Data Science Project Ideas
- 13 Data Structure Project Ideas
- 12 Real-World Python Applications
- Python Banking Project
- Data Science Course Eligibility
- Association Rule Mining Overview
- Cluster Analysis in Data Mining
- Classification in Data Mining
- KDD Process in Data Mining
- Data Structures and Algorithms
- Binary Tree Types Explained
- Binary Search Algorithm
- Sorting in Data Structure
- Binary Tree in Data Structure
- Binary Tree vs Binary Search Tree
- Recursion in Data Structure
- Data Structure Search Methods: Explained
- Binary Tree Interview Q&A
- Linear vs Binary Search
- Priority Queue Overview
- Python Programming and Tools
- Top 30 Python Pattern Programs
- List vs Tuple
- Python Free Online Course
- Method Overriding in Python
- Top 21 Python Developer Skills
- Reverse a Number in Python
- Switch Case Functions in Python
- Info Retrieval System Overview
- Reverse a Number in Python
- Real-World Python Applications
- Data Science Careers and Comparisons
- Data Analyst Salary in India
- Data Scientist Salary in India
- Free Excel Certification Course
- Actuary Salary in India
- Data Analyst Interview Guide
- Pandas Interview Guide
- Tableau Filters Explained
- Data Mining Techniques Overview
- Data Analytics Lifecycle Phases
- Data Science Vs Analytics Comparison
- Artificial Intelligence and Machine Learning Projects
- Exciting IoT Project Ideas
- 16 Exciting AI Project Ideas
- 45+ Interesting ML Project Ideas
- Exciting Deep Learning Projects
- 12 Intriguing Linear Regression Projects
- 13 Neural Network Projects
- 5 Exciting Image Processing Projects
- Top 8 Thrilling AWS Projects
- 12 Engaging AI Projects in Python
- NLP Projects for Beginners
- Concepts and Algorithms in AIML
- Basic CNN Architecture Explained
- 6 Types of Regression Models
- Data Preprocessing Steps
- Bagging vs Boosting in ML
- Multinomial Naive Bayes Overview
- Bayesian Network Example
- Bayes Theorem Guide
- Top 10 Dimensionality Reduction Techniques
- Neural Network Step-by-Step Guide
- Technical Guides and Comparisons
- Make a Chatbot in Python
- Compute Square Roots in Python
- Permutation vs Combination
- Image Segmentation Techniques
- Generative AI vs Traditional AI
- AI vs Human Intelligence
- Random Forest vs Decision Tree
- Neural Network Overview
- Perceptron Learning Algorithm
- Selection Sort Algorithm
- Career and Practical Applications in AIML
- AI Salary in India Overview
- Biological Neural Network Basics
- Top 10 AI Challenges
- Production System in AI
- Top 8 Raspberry Pi Alternatives
- Top 8 Open Source Projects
- 14 Raspberry Pi Project Ideas
- 15 MATLAB Project Ideas
- Top 10 Python NLP Libraries
- Naive Bayes Explained
- Digital Marketing Projects and Strategies
- 10 Best Digital Marketing Projects
- 17 Fun Social Media Projects
- Top 6 SEO Project Ideas
- Digital Marketing Case Studies
- Coca-Cola Marketing Strategy
- Nestle Marketing Strategy Analysis
- Zomato Marketing Strategy
- Monetize Instagram Guide
- Become a Successful Instagram Influencer
- 8 Best Lead Generation Techniques
- Digital Marketing Careers and Salaries
- Digital Marketing Salary in India
- Top 10 Highest Paying Marketing Jobs
- Highest Paying Digital Marketing Jobs
- SEO Salary in India
- Content Writer Salary Guide
- Digital Marketing Executive Roles
- Career in Digital Marketing Guide
- Future of Digital Marketing
- MBA in Digital Marketing Overview
- Digital Marketing Techniques and Channels
- 9 Types of Digital Marketing Channels
- Top 10 Benefits of Marketing Branding
- 100 Best YouTube Channel Ideas
- YouTube Earnings in India
- 7 Reasons to Study Digital Marketing
- Top 10 Digital Marketing Objectives
- 10 Best Digital Marketing Blogs
- Top 5 Industries Using Digital Marketing
- Growth of Digital Marketing in India
- Top Career Options in Marketing
- Interview Preparation and Skills
- 73 Google Analytics Interview Q&A
- 56 Social Media Marketing Q&A
- 78 Google AdWords Interview Q&A
- Top 133 SEO Interview Q&A
- 27+ Digital Marketing Q&A
- Digital Marketing Free Course
- Top 9 Skills for PPC Analysts
- Movies with Successful Social Media Campaigns
- Marketing Communication Steps
- Top 10 Reasons to Be an Affiliate Marketer
- Career Options and Paths
- Top 25 Highest Paying Jobs India
- Top 25 Highest Paying Jobs World
- Top 10 Highest Paid Commerce Job
- Career Options After 12th Arts
- Top 7 Commerce Courses Without Maths
- Top 7 Career Options After PCB
- Best Career Options for Commerce
- Career Options After 12th CS
- Top 10 Career Options After 10th
- 8 Best Career Options After BA
- Projects and Academic Pursuits
- 17 Exciting Final Year Projects
- Top 12 Commerce Project Topics
- Top 13 BCA Project Ideas
- Career Options After 12th Science
- Top 15 CS Jobs in India
- 12 Best Career Options After M.Com
- 9 Best Career Options After B.Sc
- 7 Best Career Options After BCA
- 22 Best Career Options After MCA
- 16 Top Career Options After CE
- Courses and Certifications
- 10 Best Job-Oriented Courses
- Best Online Computer Courses
- Top 15 Trending Online Courses
- Top 19 High Salary Certificate Courses
- 21 Best Programming Courses for Jobs
- What is SGPA? Convert to CGPA
- GPA to Percentage Calculator
- Highest Salary Engineering Stream
- 15 Top Career Options After Engineering
- 6 Top Career Options After BBA
- Job Market and Interview Preparation
- Why Should You Be Hired: 5 Answers
- Top 10 Future Career Options
- Top 15 Highest Paid IT Jobs India
- 5 Common Guesstimate Interview Q&A
- Average CEO Salary: Top Paid CEOs
- Career Options in Political Science
- Top 15 Highest Paying Non-IT Jobs
- Cover Letter Examples for Jobs
- Top 5 Highest Paying Freelance Jobs
- Top 10 Highest Paying Companies India
- Career Options and Paths After MBA
- 20 Best Careers After B.Com
- Career Options After MBA Marketing
- Top 14 Careers After MBA In HR
- Top 10 Highest Paying HR Jobs India
- How to Become an Investment Banker
- Career Options After MBA - High Paying
- Scope of MBA in Operations Management
- Best MBA for Working Professionals India
- MBA After BA - Is It Right For You?
- Best Online MBA Courses India
- MBA Project Ideas and Topics
- 11 Exciting MBA HR Project Ideas
- Top 15 MBA Project Ideas
- 18 Exciting MBA Marketing Projects
- MBA Project Ideas: Consumer Behavior
- What is Brand Management?
- What is Holistic Marketing?
- What is Green Marketing?
- Intro to Organizational Behavior Model
- Tech Skills Every MBA Should Learn
- Most Demanding Short Term Courses MBA
- MBA Salary, Resume, and Skills
- MBA Salary in India
- HR Salary in India
- Investment Banker Salary India
- MBA Resume Samples
- Sample SOP for MBA
- Sample SOP for Internship
- 7 Ways MBA Helps Your Career
- Must-have Skills in Sales Career
- 8 Skills MBA Helps You Improve
- Top 20+ SAP FICO Interview Q&A
- MBA Specializations and Comparative Guides
- Why MBA After B.Tech? 5 Reasons
- How to Answer 'Why MBA After Engineering?'
- Why MBA in Finance
- MBA After BSc: 10 Reasons
- Which MBA Specialization to choose?
- Top 10 MBA Specializations
- MBA vs Masters: Which to Choose?
- Benefits of MBA After CA
- 5 Steps to Management Consultant
- 37 Must-Read HR Interview Q&A
- Fundamentals and Theories of Management
- What is Management? Objectives & Functions
- Nature and Scope of Management
- Decision Making in Management
- Management Process: Definition & Functions
- Importance of Management
- What are Motivation Theories?
- Tools of Financial Statement Analysis
- Negotiation Skills: Definition & Benefits
- Career Development in HRM
- Top 20 Must-Have HRM Policies
- Project and Supply Chain Management
- Top 20 Project Management Case Studies
- 10 Innovative Supply Chain Projects
- Latest Management Project Topics
- 10 Project Management Project Ideas
- 6 Types of Supply Chain Models
- Top 10 Advantages of SCM
- Top 10 Supply Chain Books
- What is Project Description?
- Top 10 Project Management Companies
- Best Project Management Courses Online
- Salaries and Career Paths in Management
- Project Manager Salary in India
- Average Product Manager Salary India
- Supply Chain Management Salary India
- Salary After BBA in India
- PGDM Salary in India
- Top 7 Career Options in Management
- CSPO Certification Cost
- Why Choose Product Management?
- Product Management in Pharma
- Product Design in Operations Management
- Industry-Specific Management and Case Studies
- Amazon Business Case Study
- Service Delivery Manager Job
- Product Management Examples
- Product Management in Automobiles
- Product Management in Banking
- Sample SOP for Business Management
- Video Game Design Components
- Top 5 Business Courses India
- Free Management Online Course
- SCM Interview Q&A
- Fundamentals and Types of Law
- Acceptance in Contract Law
- Offer in Contract Law
- 9 Types of Evidence
- Types of Law in India
- Introduction to Contract Law
- Negotiable Instrument Act
- Corporate Tax Basics
- Intellectual Property Law
- Workmen Compensation Explained
- Lawyer vs Advocate Difference
- Law Education and Courses
- LLM Subjects & Syllabus
- Corporate Law Subjects
- LLM Course Duration
- Top 10 Online LLM Courses
- Online LLM Degree
- Step-by-Step Guide to Studying Law
- Top 5 Law Books to Read
- Why Legal Studies?
- Pursuing a Career in Law
- How to Become Lawyer in India
- Career Options and Salaries in Law
- Career Options in Law India
- Corporate Lawyer Salary India
- How To Become a Corporate Lawyer
- Career in Law: Starting, Salary
- Career Opportunities: Corporate Law
- Business Lawyer: Role & Salary Info
- Average Lawyer Salary India
- Top Career Options for Lawyers
- Types of Lawyers in India
- Steps to Become SC Lawyer in India
- Tutorials
- Software Tutorials
- C Tutorials
- Recursion in C: Fibonacci Series
- Checking String Palindromes in C
- Prime Number Program in C
- Implementing Square Root in C
- Matrix Multiplication in C
- Understanding Double Data Type
- Factorial of a Number in C
- Structure of a C Program
- Building a Calculator Program in C
- Compiling C Programs on Linux
- Java Tutorials
- Handling String Input in Java
- Determining Even and Odd Numbers
- Prime Number Checker
- Sorting a String
- User-Defined Exceptions
- Understanding the Thread Life Cycle
- Swapping Two Numbers
- Using Final Classes
- Area of a Triangle
- Skills
- Explore Skills
- Management Skills
- Software Engineering
- JavaScript
- Data Structure
- React.js
- Core Java
- Node.js
- Blockchain
- SQL
- Full stack development
- Devops
- NFT
- BigData
- Cyber Security
- Cloud Computing
- Database Design with MySQL
- Cryptocurrency
- Python
- Digital Marketings
- Advertising
- Influencer Marketing
- Performance Marketing
- Search Engine Marketing
- Email Marketing
- Content Marketing
- Social Media Marketing
- Display Advertising
- Marketing Analytics
- Web Analytics
- Affiliate Marketing
- MBA
- MBA in Finance
- MBA in HR
- MBA in Marketing
- MBA in Business Analytics
- MBA in Operations Management
- MBA in International Business
- MBA in Information Technology
- MBA in Healthcare Management
- MBA In General Management
- MBA in Agriculture
- MBA in Supply Chain Management
- MBA in Entrepreneurship
- MBA in Project Management
- Management Program
- Consumer Behaviour
- Supply Chain Management
- Financial Analytics
- Introduction to Fintech
- Introduction to HR Analytics
- Fundamentals of Communication
- Art of Effective Communication
- Introduction to Research Methodology
- Mastering Sales Technique
- Business Communication
- Fundamentals of Journalism
- Economics Masterclass
- Free Courses
- Home
- Blog
- Data Science
- Sorting in Data Structure: Categories & Types [With Examples]
Sorting in Data Structure: Categories & Types [With Examples]
Updated on Dec 27, 2024 | 22 min read
Share:
Table of Contents
- Why is Sorting Important in Data Structures?
- 10 Top Algorithms of Sorting in Data Structures Explained
- Time and Space Complexity of Sorting Algorithms
- Sorting Algorithm Characteristics: Stability, In-Place, and Adaptiveness
- Practical Applications of Sorting Algorithms in Real Life
- How to Choose the Right Sorting in Data Structure Algorithm
- Sorting Algorithm Cheat Sheet for Data Science
Sorting in data structures is the process of arranging data in a specific order, usually ascending or descending. Sorting makes data easier to navigate and improves the efficiency of tasks like searching and analysis. Think of a dictionary—without alphabetical order, finding a word would be difficult and time-consuming. For data professionals, sorting organizes raw data into a structured format, making it manageable and accessible.
Without sorting, handling large datasets becomes challenging, slowing down essential operations. Sorting brings clarity and structure, allowing faster access to key information.
In this post, we’ll explore the main types of sorting algorithms in data structures. First, let’s look at what a sorting algorithm is and its role in data organization.
- What is Sorting in Data Structure?: A set of steps that arranges elements in a specific order, starting with an unsorted array and resulting in an ordered sequence.
- Example: Input array: [h, j, k, i, n, m, o, l]
Sorted output: [h, i, j, k, l, m, n, o]
Continue reading as we cover the types of sorting algorithms, categories, and their role in data structure management.
Sorting makes life easier. Explore our data science courses to learn more about data science algorithms. Check out now!
Why is Sorting Important in Data Structures?
Sorting in data structure is fundamental because it directly impacts the performance of search and indexing operations. In a sorted dataset, algorithms like binary search can be applied, reducing search time complexity from O(n) to O(log n). Sorting is also essential for efficient data processing in areas like ranking, priority-based retrieval, and merging data.
Checkout: Types of Binary Tree
Key Technical Benefits:
- Enhanced Search Efficiency: Sorted data enables binary search, significantly reducing search time.
- Improved Data Access: Operations like finding the minimum, maximum, or median are faster with sorted data.
- Basis for Complex Algorithms: Sorting is required for several advanced techniques, such as divide-and-conquer strategies and tree-based structures, where ordered data is necessary.
Sorting ensures that data is organized, making it accessible and efficient for further operations in data processing and analysis.
Sorting Categories: Internal vs External Sorting
Sorting algorithms are classified into internal sorting and external sorting based on data size and memory constraints.
Sorting Type |
Definition |
Optimal Use Case |
Common Algorithms |
Internal Sorting |
Sorting done entirely in RAM. |
Suitable for small to medium datasets. |
Quick Sort, Merge Sort, Heap Sort |
External Sorting |
Sorting that requires external storage, usually disk-based. |
Used for large datasets that exceed memory. |
External Merge Sort, Multi-way Merge Sort |
Internal Sorting
Internal sorting in data structure is used when the entire dataset can fit within main memory (RAM), allowing for faster processing without requiring external storage access.
- Characteristics:
- Memory-Based Processing: Works entirely in RAM, which is faster than accessing external storage.
- Best for Small to Medium Datasets: Suitable when data size does not exceed available memory.
- Common Algorithms:
- Quick Sort: An in-place algorithm with average-case complexity of O(n log n). Effective but has a worst-case time complexity of O(n²) if the pivot selection is poor.
- Merge Sort: A stable algorithm with O(n log n) complexity, requiring additional memory for merging.
- Heap Sort: Uses a binary heap structure to achieve O(n log n) complexity, often used in priority-based sorting tasks.
Typical Use: Internal sorting is used in applications where data fits in memory, such as sorting lists or arrays in moderate-sized datasets.
External Sorting
External sorting in data structure is required when the dataset is too large to fit into main memory and must use external storage for sorting operations.
- Characteristics:
- Disk-Based Processing: Requires data to be read from and written to external storage, impacting performance.
- Best for Large Datasets: Necessary when data exceeds memory capacity, such as in large-scale data processing.
- Common Algorithms:
- External Merge Sort: Splits data into manageable chunks, sorts each chunk in memory, and merges them. Efficient for large datasets, with a time complexity of O(n log n).
- Multi-way Merge Sort: Extends merge sort to sort and merge multiple chunks simultaneously, reducing the number of I/O operations.
Typical Use: Used in systems managing large data files, such as database systems, where sorting needs exceed available RAM.
Categories of Sorting Algorithms: Comparison vs Non-Comparison Sorting
Sorting algorithms are categorized into comparison-based and non-comparison-based types, each with specific technical characteristics suited for different data structures and application requirements.
Category |
Definition |
Examples |
Optimal Use Case |
Comparison-based Sorting |
Uses direct element comparisons, O(n log n) limit. |
Bubble Sort, Quick Sort, Merge Sort, Heap Sort |
Flexible, general-purpose sorting on datasets where comparisons are feasible. |
Non-Comparison-based Sorting |
Bypasses comparisons, uses other properties for sorting, achieving O(n) in constrained cases. |
Counting Sort, Radix Sort, Bucket Sort |
Ideal for large datasets with known structures, achieving linear time in specific scenarios. |
Comparison-based Sorting Algorithms
Comparison-based sorting algorithms determine order by comparing elements directly, with a lower bound time complexity of O(n log n) due to comparison limits in sorting.
- Examples:
- Bubble Sort: Uses adjacent comparisons and swaps, O(n²) time complexity, inefficient on large datasets but stable.
- Quick Sort: Recursive, in-place, average O(n log n) with O(n²) worst case; relies on pivot selection for optimal performance.
- Merge Sort: Stable, O(n log n) complexity, memory-intensive due to merging, making it effective for linked lists.
- Heap Sort: Constructs a max-heap, sorts in O(n log n), suitable for priority sorting without additional memory.
Use Cases:
- General-purpose sorting where elements can be directly compared.
- Effective on data types with defined comparison operators.
- Ideal for in-memory datasets with moderate size.
Non-Comparison-based Sorting Algorithms
Non-comparison sorting algorithms bypass element comparisons, using techniques like digit processing or counting, achieving linear time complexity under specific conditions.
- Examples:
- Counting Sort: Uses frequency counts; O(n + k) complexity, where k is value range, best for small integer ranges.
- Radix Sort: Processes digits from least to most significant; O(d * (n + b)) complexity, where d is digit count and b is base, ideal for fixed-length integers or strings.
- Bucket Sort: Divides data into "buckets"; O(n) complexity in the best case, efficient for uniformly distributed data.
Use Cases:
- Optimal for data with known characteristics like limited ranges or digit-based values.
- Achieves linear time for specific cases (e.g., integer or string sorting).
- Commonly used for large datasets with restricted value ranges.
10 Top Algorithms of Sorting in Data Structures Explained
For each algorithm, you’ll find a clear explanation, practical examples, and Python code to see them in action.
1. Bubble Sort Algorithm: Step-by-Step Process
How it Works:
Bubble Sort is a simple, comparison-based algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Each pass through the list places the next-largest element in its correct position, “bubbling” the largest unsorted elements to the end of the list.
- Time Complexity: O(n²) in the average and worst cases, due to nested loops comparing and swapping each element.
- Space Complexity: O(1), as Bubble Sort operates in place without requiring additional memory.
- Characteristics: Stable, meaning it preserves the order of duplicate elements, but inefficient for large datasets due to quadratic time complexity.
Example Use Case:
Bubble Sort is often used in educational contexts to demonstrate sorting fundamentals. In practice, it may be used in scenarios with small, nearly sorted datasets where simplicity is prioritized over efficiency.
Step-by-Step Example: Given an input array [6, 3, 7, 1, 2, 4]:
- First Pass:
- Compare 6 and 3; swap → [3, 6, 7, 1, 2, 4]
- Compare 6 and 7; no swap → [3, 6, 7, 1, 2, 4]
- Compare 7 and 1; swap → [3, 6, 1, 7, 2, 4]
- Continue until the largest element “bubbles” to the end.
Python Code:
def bubbleSort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example
arr = [64, 34, 25, 12, 22, 11, 90]
print("Sorted array is:", bubbleSort(arr))
2. Quick Sort: Fastest Sorting for Large Datasets
How it Works:
Quick Sort is a divide-and-conquer algorithm that selects a “pivot” element and partitions the array around the pivot. Elements smaller than the pivot are placed on its left, and elements greater than the pivot on its right. This partitioning continues recursively until the array is fully sorted.
- Time Complexity: O(n log n) on average. Worst case is O(n²), occurring when the smallest or largest element is always chosen as the pivot.
- Space Complexity: O(log n) due to recursive stack calls in an ideal scenario.
- Characteristics: Quick Sort is not stable but is highly efficient for large datasets due to its low average complexity and in-place sorting.
Example Use Case:
Quick Sort is ideal for large datasets and is commonly used in applications like search engines and database sorting where fast sorting is required.
Step-by-Step Example: For an array [6, 3, 7, 1, 2, 4], using 4 as the pivot:
- Partitioning: Elements less than 4 ([3, 1, 2]) and elements greater than 4 ([6, 7]).
- Recursively apply Quick Sort on each partition until the entire array is sorted.
Python Code:
def quickSort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quickSort(left) + middle + quickSort(right)
# Example
arr = [3, 6, 8, 10, 1, 2, 1]
print("Sorted array is:", quickSort(arr))
3. Merge Sort: Efficient for External Sorting
How it Works:
Merge Sort is a stable, divide-and-conquer algorithm that recursively divides the array into two halves, sorts each half, and merges the sorted halves back together. It’s highly efficient for sorting linked lists or external sorting in database systems, as it maintains stable ordering.
- Time Complexity: O(n log n) in all cases due to consistent halving and merging operations.
- Space Complexity: O(n), as it requires additional memory for merging sorted halves.
- Characteristics: Stable sorting, which maintains the relative order of duplicate elements, making it suitable for datasets where order preservation is important.
Example Use Case:
Merge Sort is preferred for large data stored on external devices, such as in database systems where merging sorted chunks of data is required.
Step-by-Step Example: For an array [6, 3, 7, 1, 2, 4]:
- Split into [6, 3, 7] and [1, 2, 4].
- Sort each half recursively, then merge the sorted halves into [1, 2, 3, 4, 6, 7].
Python Code:
def mergeSort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
mergeSort(L)
mergeSort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
# Example
arr = [12, 11, 13, 5, 6, 7]
mergeSort(arr)
print("Sorted array is:", arr)
4. Heap Sort: Memory-Efficient Sorting
How it Works:
Heap Sort is an efficient, comparison-based algorithm that builds a max heap from the input data. It repeatedly removes the root (maximum) element from the heap, places it at the end of the array, and “heapifies” the remaining elements. This process continues until the array is fully sorted.
- Steps:
- Build a Max Heap from the input data.
- Extract the Maximum Element (root) and place it at the end of the array.
- Heapify the Remaining Elements to maintain the max heap structure.
- Repeat until all elements are sorted.
- Time Complexity: O(n log n) for all cases, as heapifying each element takes log n time and is repeated for each element.
- Space Complexity: O(1), as Heap Sort is an in-place sorting algorithm and doesn’t require additional memory.
- Example: Used in applications like priority queue management, where items need to be retrieved based on priority order.
Python Code:
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[i] < arr[left]:
largest = left
if right < n and arr[largest] < arr[right]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heapSort(arr):
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n-1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
# Example
arr = [4, 10, 3, 5, 1]
heapSort(arr)
print("Sorted array is:", arr)
5. Counting Sort: Best for Limited Range Data
How it Works:
Counting Sort works by counting the occurrences of each distinct element in the input array and storing these counts in an auxiliary array. The sorted array is then constructed by using the cumulative counts to place each element in its correct position.
- Steps:
- Count Occurrences: Count each element and store it in the count array.
- Calculate Cumulative Counts: Modify the count array to reflect the positions of each element.
- Build the Sorted Array: Use the cumulative count array to place elements in the sorted order.
- Time Complexity: O(n + k), where n is the number of elements and k is the range of the input.
- Space Complexity: O(k), as additional memory is needed for the count array.
- Example: Suitable for applications with fixed, limited-range data, such as sorting grades, where the data values fall within a specific range.
Python Code:
def countingSort(arr):
max_val = max(arr)
count = [0] * (max_val + 1)
output = [0] * len(arr)
for num in arr:
count[num] += 1
for i in range(1, len(count)):
count[i] += count[i - 1]
for num in reversed(arr):
output[count[num] - 1] = num
count[num] -= 1
return output
# Example
arr = [4, 2, 2, 8, 3, 3, 1]
sorted_arr = countingSort(arr)
print("Sorted array is:", sorted_arr)
6. Radix Sort: Sorting Numbers by Their Digits
How it Works:
Radix Sort sorts integers by processing each digit individually, starting from the least significant digit to the most significant digit. It uses Counting Sort as a subroutine to sort numbers based on each digit, one place at a time.
- Steps:
- Sort by Least Significant Digit: Sort the numbers based on their least significant digit (units).
- Proceed to the Next Digit: Move to the next digit and repeat the sorting process.
- Repeat for All Digits: Continue until all digit places are processed, resulting in a fully sorted array.
- Time Complexity: O(nk), where n is the number of elements and k is the maximum number of digits.
- Space Complexity: O(n + k), due to the additional memory used in Counting Sort for each digit.
- Example: Ideal for sorting large numbers or strings with a fixed digit length, often used in applications needing digit-based sorting.
Python Code:
def countingSortForRadix(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
for i in range(n - 1, -1, -1):
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
for i in range(n):
arr[i] = output[i]
def radixSort(arr):
max_val = max(arr)
exp = 1
while max_val // exp > 0:
countingSortForRadix(arr, exp)
exp *= 10
# Example
arr = [170, 45, 75, 90, 802, 24, 2, 66]
radixSort(arr)
print("Sorted array is:", arr)
7. Selection Sort: Simple but Inefficient
How it Works:
Selection Sort divides the array into a sorted and an unsorted region. It repeatedly searches for the smallest element in the unsorted portion and swaps it with the leftmost unsorted element, gradually building a sorted list on the left side of the array.
- Steps:
- Find the Minimum Element in the unsorted part of the array.
- Swap the minimum element with the leftmost unsorted element.
- Move the Boundary between sorted and unsorted sections by one position to the right.
- Repeat until the entire array is sorted.
- Time Complexity: O(n²) in all cases, as it performs O(n) searches for each element.
- Space Complexity: O(1), as it is an in-place algorithm requiring no additional memory.
- Example: Selection Sort is best suited for small datasets where memory efficiency is critical but time efficiency is not a primary concern.
Python Code:
def selectionSort(arr):
for i in range(len(arr)):
min_idx = i
for j in range(i+1, len(arr)):
if arr[min_idx] > arr[j]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
# Example
arr = [6, 25, 10, 28, 11]
selectionSort(arr)
print("Sorted array is:", arr)
8. Insertion Sort: Best for Nearly Sorted Data
How it Works:
Insertion Sort is an adaptive, comparison-based algorithm that builds the sorted array one element at a time by inserting each new element into its correct position within the sorted portion. This is similar to the way people organize playing cards by picking one card at a time and placing it in the correct order with the already-sorted cards.
- Steps:
- Start from the second element and treat the first element as sorted.
- Compare the current element with the elements in the sorted part of the array, moving from right to left.
- Shift Elements to the right to make space for the current element if it’s smaller.
- Insert the current element in its correct position within the sorted portion.
- Repeat until all elements are placed in order.
- Time Complexity: O(n²) in the average and worst cases, but O(n) in the best case for nearly sorted data.
- Space Complexity: O(1), as it is an in-place algorithm.
- Example: Insertion Sort is ideal for nearly sorted data or small datasets where fast insertion is needed. Its adaptive nature makes it efficient for datasets that are already close to being sorted.
Python Code:
def insertionSort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
# Example
arr = [12, 11, 13, 5, 6]
insertionSort(arr)
print("Sorted array is:", arr)
9. Bucket Sort: Grouping Data into Buckets
How it Works:
Bucket Sort works by dividing the dataset into a set number of “buckets” based on a specific range. Each bucket holds elements that fall within a particular range. After distributing elements into buckets, each bucket is sorted individually, often using another sorting algorithm like Insertion Sort. Finally, the sorted buckets are combined to form the complete sorted array.
- Steps:
- Create Buckets: Initialize an empty array of buckets, each representing a specific range.
- Distribute Elements: Place each element into the appropriate bucket based on its value.
- Sort Individual Buckets: Sort each bucket using an appropriate sorting algorithm (usually Insertion Sort).
- Concatenate Buckets: Combine the sorted elements from each bucket into the final sorted array.
- Time Complexity: O(n + k), where n is the number of elements and k is the number of buckets. The complexity depends on how evenly distributed the data is and the sorting method within each bucket.
- Space Complexity: O(n), as it requires additional memory for the buckets.
- Example: Bucket Sort is commonly used for sorting uniformly distributed data, such as floating-point numbers between 0 and 1 or data with values that fit within a known range.
Python Code:
def bucketSort(arr):
bucket_count = 10
max_value = max(arr)
size = max_value / bucket_count
buckets = [[] for _ in range(bucket_count)]
for num in arr:
bucket_index = int(num / size)
if bucket_index != bucket_count:
buckets[bucket_index].append(num)
else:
buckets[bucket_count - 1].append(num)
for bucket in buckets:
bucket.sort()
sorted_arr = []
for bucket in buckets:
sorted_arr.extend(bucket)
return sorted_arr
# Example
arr = [0.78, 0.17, 0.39, 0.26, 0.72, 0.94, 0.21, 0.12, 0.23, 0.68]
sorted_arr = bucketSort(arr)
print("Sorted array is:", sorted_arr)
10. Shell Sort: Improved Insertion Sort
How it Works:
Shell Sort is an enhanced version of Insertion Sort that improves sorting efficiency by first sorting elements far apart, gradually reducing the gap until it becomes 1 (like Insertion Sort). By using a decreasing gap sequence, Shell Sort allows elements to be moved closer to their correct position faster, reducing the total number of shifts needed.
- Steps:
- Choose a Gap Sequence: Determine an initial gap, typically half the array length, and reduce it progressively.
- Sort with the Current Gap: For each gap, compare and sort elements separated by this distance.
- Reduce the Gap: Continue reducing the gap until it reaches 1, performing Insertion Sort in the final iteration.
- Time Complexity: O(n log n) in the best cases, but varies based on the gap sequence. Common sequences include Shell’s original sequence and Hibbard’s sequence.
- Space Complexity: O(1), as Shell Sort is an in-place algorithm.
- Example: Shell Sort is effective for medium-sized datasets and is particularly useful when dealing with datasets that are already partially sorted.
Python Code:
def shellSort(arr):
n = len(arr)
gap = n // 2
while gap > 0:
for i in range(gap, n):
temp = arr[i]
j = i
while j >= gap and arr[j - gap] > temp:
arr[j] = arr[j - gap]
j -= gap
arr[j] = temp
gap //= 2
# Example
arr = [12, 34, 54, 2, 3]
shellSort(arr)
print("Sorted array is:", arr)
Curious about Data Frames in Python? Check out our in-depth tutorial and level up your Python skills today!
Time and Space Complexity of Sorting Algorithms
Sorting in data structures comes with challenges that affect speed, efficiency, and memory use, especially with large datasets.
What is Time Complexity?
Time Complexity is a measure of the amount of time an algorithm takes to complete as a function of the input size (n). It reflects the number of operations an algorithm performs and is generally expressed in Big O notation. Time complexity helps in understanding the efficiency of an algorithm across different scenarios:
- Best Case: The minimum time the algorithm requires (often with optimally arranged input).
- Average Case: The expected time for a typical input, showing general performance.
- Worst Case: The maximum time the algorithm may take, which provides an upper bound for performance.
What is Space Complexity?
Space Complexity is a measure of the amount of memory an algorithm requires during execution. This includes memory needed for input, auxiliary variables, and any additional data structures. Like time complexity, space complexity is usually expressed in Big O notation. It helps in evaluating how memory-efficient an algorithm is, especially when working with memory constraints.
This table provides a comparison of time complexity (best, worst, and average cases) and space complexity for each commonly used sorting algorithm. Examples are included to highlight scenarios where each algorithm performs best based on data size and memory considerations.
Sorting Algorithm |
Best Case |
Average Case |
Worst Case |
Space Complexity |
Example Use Cases |
Bubble Sort |
O(n) |
O(n²) |
O(n²) |
O(1) |
Small datasets or nearly sorted data where simplicity is prioritized over speed. |
Selection Sort |
O(n²) |
O(n²) |
O(n²) |
O(1) |
Small datasets where memory is limited but time efficiency is not critical. |
Insertion Sort |
O(n) |
O(n²) |
O(n²) |
O(1) |
Nearly sorted or small datasets where adaptive performance is needed. |
Merge Sort |
O(n log n) |
O(n log n) |
O(n log n) |
O(n) |
Large datasets, especially for external sorting (e.g., databases) requiring stable sorting. |
Quick Sort |
O(n log n) |
O(n log n) |
O(n²) |
O(log n) |
Large, unsorted datasets where speed is crucial, such as in search engines. |
Heap Sort |
O(n log n) |
O(n log n) |
O(n log n) |
O(1) |
Priority queues or large datasets where in-place sorting is required. |
Counting Sort |
O(n + k) |
O(n + k) |
O(n + k) |
O(k) |
Fixed-range integer data, such as grading or categorizing data by ranges. |
Radix Sort |
O(nk) |
O(nk) |
O(nk) |
O(n + k) |
Sorting large numbers or data with a fixed digit length. |
Bucket Sort |
O(n) |
O(n) |
O(n²) |
O(n) |
Uniformly distributed data, like decimal numbers between 0 and 1. |
Shell Sort |
O(n log n) |
Varies (depends on gap sequence) |
Varies (depends on gap sequence) |
O(1) |
Medium-sized datasets that are already partially sorted. |
Sorting Algorithm Characteristics: Stability, In-Place, and Adaptiveness
Stability, in-place sorting, and adaptiveness can help choose the right sorting algorithm based on data requirements.
- Stability: A stable sorting algorithm preserves the relative order of elements with equal values. Stability is particularly important when sorting data with multiple keys, as it ensures that previous orderings are retained.
- Stable Algorithms: Bubble Sort, Insertion Sort, Merge Sort, Counting Sort, Radix Sort.
- Non-Stable Algorithms: Quick Sort, Heap Sort, Selection Sort.
- In-Place Sorting: An in-place algorithm sorts the array without requiring additional memory proportional to the input size. In-place sorting is memory-efficient and preferred when working within strict memory constraints.
- In-Place Algorithms: Quick Sort, Heap Sort, Bubble Sort, Selection Sort, Insertion Sort.
- Not In-Place Algorithms: Merge Sort, Counting Sort, Radix Sort, Bucket Sort (require extra memory).
- Adaptive Sorting: An adaptive algorithm improves its performance when the data is already partially sorted. This characteristic reduces the time complexity in nearly sorted arrays, making these algorithms efficient in such cases.
- Adaptive Algorithms: Bubble Sort, Insertion Sort, and (depending on gap sequence) Shell Sort.
- Non-Adaptive Algorithms: Merge Sort, Quick Sort, Heap Sort, Selection Sort.
Practical Applications of Sorting Algorithms in Real Life
Sorting algorithms are everywhere in real life, and help organize data for faster access and better management. Here are a few examples:
- Quick Sort and Merge Sort help search engines quickly organize and retrieve vast amounts of data. Quick Sort is fast for partitioning data, while Merge Sort is great for handling large volumes of information reliably.
- Sorting is essential for organizing transactions by time, amount, or account number. It helps banks and financial services keep records in order, track trades, and create time-based reports.
- Databases use sorting to speed up data access by organizing records for faster searches. Sorting data in databases is essential for smooth, quick queries and easy access to stored information.
- Sorting helps inventory systems and online stores organize products by price, popularity, or category. This makes it easier for customers to find what they need quickly.
- Sorting keeps data packets organized in network systems and files in operating systems, making data access faster and more efficient.
How to Choose the Right Sorting in Data Structure Algorithm
Here’s a quick guide:
- Dataset Size:
- Small Datasets: Insertion Sort or Selection Sort work well for small datasets because they’re simple and don’t require much memory.
- Large Datasets: Quick Sort and Merge Sort are better for large datasets. Quick Sort is fast and efficient for in-place sorting, while Merge Sort is a good choice for large datasets that need stable sorting.
- Time Constraints:
- Real-Time Needs: Quick Sort is a great choice when results are needed quickly, as it’s one of the fastest sorting methods.
- Consistency: Merge Sort is more predictable, making it ideal when you need reliable sorting times.
- Memory Availability:
- Limited Memory: Heap Sort or in-place Quick Sort are best when memory is tight, as they don’t need much extra space.
- More Memory Available: Merge Sort and Counting Sort are ideal when extra memory is available, as they use this to make sorting more stable and efficient.
Examples:
- Quick Sort is ideal for search engines or apps that need fast sorting.
- Merge Sort works well in e-commerce or databases where stable sorting is important.
Sorting Algorithm Cheat Sheet for Data Science
Sorting algorithms help organize and process data efficiently. upGrad’s data science courses can teach you to apply them in real-world projects.
Check them out to build hands-on skills for your career.
Quick Sort
- Best For: Large datasets needing quick sorting.
- Key Feature: Uses a pivot for fast, efficient sorting.
- upGrad Advantage: Learn Quick Sort in real data projects with upGrad’s data science courses.
Merge Sort
- Best For: Large datasets where stability matters.
- Key Feature: Uses a divide-and-conquer method for consistent results.
- upGrad Advantage: Master Merge Sort with hands-on projects on upGrad.
Heap Sort
- Best For: Situations with limited memory.
- Key Feature: Memory-efficient sorting using binary heaps.
- upGrad Advantage: Get guidance on Heap Sort in memory-constrained settings through upGrad’s expert-led sessions.
Counting Sort
- Best For: Fixed-range data like grades or IDs.
- Key Feature: Non-comparison sorting that handles limited values efficiently.
- upGrad Advantage: Explore non-comparative sorting with upGrad’s data science modules.
Build your skills with upGrad’s data science courses. Learn essential sorting algorithms and gain real-world experience. Start today!
Ready to become a data expert? Check out our Online Data Science Courses!
Learn from top industry professionals and see your career progress in this high-demand field. Start your journey today!
Enhance your expertise with our Data Science Courses. Explore the programs below to find your perfect fit.
Explore our Popular Data Science Courses
Elevate your sought-after data science skills with our leading programs. Explore the perfect course for your goals below.
Top Data Science Skills to Learn
Discover trending articles on data science to deepen your knowledge. Browse the resources below to find your ideal match.
Read our popular Data Science Articles
Frequently Asked Questions (FAQs)
1. What is the most efficient sorting algorithm for large datasets?
2. When should I use Merge Sort instead of Quick Sort?
3. What are in-place sorting algorithms?
4. Which sorting algorithm is best for memory-limited environments?
5. What does it mean for a sorting algorithm to be stable?
6. Can sorting algorithms be used in real-time systems?
7. Why is Bubble Sort rarely used in real-world applications?
8. How does sorting affect search algorithms like binary search?
9. What is the difference between adaptive and non-adaptive sorting algorithms?
10. How can I practice implementing sorting algorithms?
11. What are the benefits of non-comparison sorting algorithms like Counting Sort?
Get Free Consultation
By submitting, I accept the T&C and
Privacy Policy
Start Your Career in Data Science Today
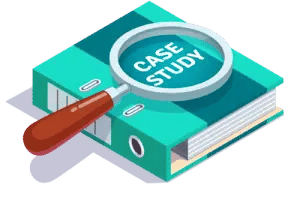
Top Resources